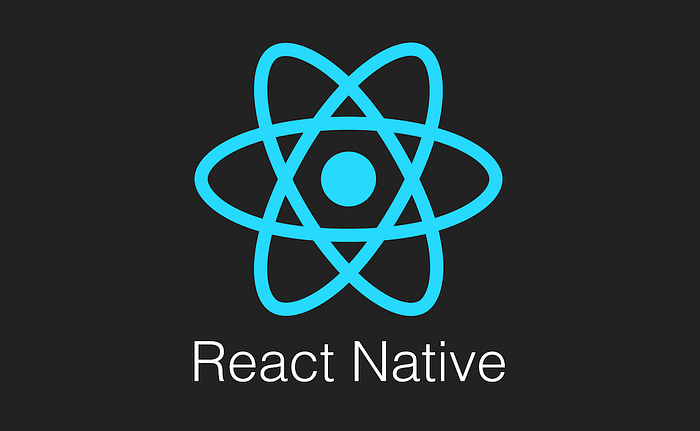
When you’re looking to develop mobile applications efficiently, React Native is one of the best frameworks out there. React Native allows developers to write code that works seamlessly on both iOS and Android platforms, making it a cost-effective choice. In this guide, we’ll show you how to build a simple calculator app using React Native, which can help you get hands-on experience with this popular framework.
Whether you’re a beginner or an experienced developer, building a calculator app will teach you some key concepts of React Native like handling user input, managing state, and displaying dynamic results. So, let’s dive right into it and start building your first React Native app!
What Is React Native?
React Native is a JavaScript framework for building native mobile applications. It allows developers to write code that works on both Android and iOS platforms using the same codebase. The framework is based on React, which is a popular JavaScript library for building user interfaces.
What sets React Native apart from other frameworks is its ability to use native components, such as buttons, sliders, and text inputs, which gives the apps built with React Native a truly native feel. The ability to reuse code and have access to native APIs makes React Native an excellent choice for cross-platform development.
Setting Up Your Development Environment
Before you can start building your calculator app with React Native, you need to set up your development environment. Here are the necessary steps:
-
Install Node.js: React Native relies on Node.js for package management. Download and install Node.js from the official website.
-
Install React Native CLI: Open your terminal and run the following command to install React Native CLI globally:
-
Install Android Studio or Xcode: Depending on whether you’re developing for Android or iOS, you’ll need to set up either Android Studio (for Android apps) or Xcode (for iOS apps).
-
Create a New React Native Project: Once you have all the necessary tools set up, you can create a new React Native project by running the following command in your terminal:
This will create a new directory with the basic files needed to start your project.
Building the Calculator App UI
Now that your development environment is ready, let’s start building the user interface (UI) for your calculator app. The main components of the calculator will include buttons for numbers and operations, and a display area to show the results.
-
Creating the Button Components:
Start by defining the calculator’s buttons. In React Native, buttons are typically created using the
TouchableOpacity
component, which allows you to make buttons that can respond to user interactions.Here’s a simple example of a button component:
This code defines a reusable button component that you can use for each calculator button.
-
Layout for Calculator Display and Buttons:
Next, let’s create a layout for your app. You will need a
TextInput
component to display the input and result, as well as buttons for numbers and operations. Here’s an example of the layout code:This code sets up the basic layout with buttons arranged in rows and a display area at the top. The buttons include numbers, basic operators, and a ‘C’ button to clear the input.
Handling User Input and Operations
Now that we’ve set up the UI, it’s time to add functionality to the app. The useState
hook in React Native helps us manage the state of the input. We also need to handle basic mathematical operations.
-
Handling Button Presses:
Each button press updates the state of the input field. For operators like addition and subtraction, we append them to the input string. For the “=” button, we evaluate the expression using JavaScript’s
eval()
function. -
Evaluating Expressions:
When the “=” button is pressed, the input string is evaluated as a mathematical expression. If there is an error (e.g., dividing by zero), we catch it and display “Error” in the input field.
Testing the App
Once the app is complete, it’s important to test it thoroughly to ensure it works as expected. Here are some tests to run:
- Pressing numbers should update the display correctly.
- Pressing operators (+, -, *, /) should append them to the input string.
- Pressing “=” should evaluate the expression and show the result.
- Pressing “C” should clear the display.
Conclusion
In this guide, we’ve shown you how to build a simple calculator app using React Native. By walking through each step, from setting up your development environment to implementing the app’s functionality, you’ve gained a better understanding of how React Native works. Not only did you learn how to create an app, but you also learned about handling user input, managing state, and building an interactive UI.
Building small apps like a calculator is a great way to hone your skills with React Native. It teaches you foundational concepts that you can apply to larger projects. As you become more comfortable with React Native, you’ll be able to create even more complex apps that run seamlessly on both Android and iOS devices.